WHAT IS THIS
The programming language used to write this code is javascript , and used here to write instructions to be included in a web page, which are to be interpreted by the browser. The operation performed by this script is to show on a html page a succession of images with a fading effect .
To do this we need the following elements
1) we have to give the images a name with a number in succession
2) the style sheet is quite easy
3) the javascript
is
@charset "utf-8";
/* CSS Document */
#slideshow {
border: 0px solid #000;
overflow: hidden;
max-height: 500px; max-width: 700px;
margin-top: 0px;margin-bottom: 0px;
margin-left: auto;
margin-right: auto;
}
#slideshow img {
width: 700px;
height:500px;
overflow: hidden;
border-radius:20px;
} |
This file, saved as slideshow..css gives the following instructions to the interpreter:
- @charset "utf-8" that must be used as the first instruction to give the character set
- #slideshow { It indicates that formatting concerns a div named slideshow that will be set into the body of the html page. The brace indicates the beginning of the instructions. and the whole is close with a bracket }
- Other istructions we set the slideshow border (0, no border), the maximum height and width of the slideshow the margins top and bottom, (both = 0) and the other margins to automatic..Remember that the end of a single instruction is marked by ;
- #slideshow_img we set the image's width and height ,and also want the corner rounding, and set it to 20px. Equal for all corners.
JAVASCRIPT FOR THE SLIDESHOW
A little bit more complicate is to explain how the javascript is done because one should know the language to understand it. Let me try. This script consists of 3 parts , that are
1) the listener waiting for the page loading event
2) the variable declarations that is the css and the images array
3) the function so_init that use the variables declarations and displays the images for a given time
4) the function so _xfade, that operates the image fading and it's launched by so_ init
/*This add a so called 'event listener' that run the event when the page is loaded. starting the function so_init */
window.addEventListener?window.addEventListener('load',so_init,false):window.attachEvent('onload',so_init);
/*This declares a variable d and an array named imgs to contaIn the images list.*//*
var d=document, imgs = new Array(), zInterval = null, current=0, pause=false;
function so_init()
{
if(!d.getElementById || !d.createElement)return;
/*This creates a link to the following css only if the css is not available and places it in the variable d *//*
css = d.createElement('link');
css.setAttribute('href','slideshow.css');
css.setAttribute('rel','stylesheet');
css.setAttribute('type','text/css');
/*This append the element css created in the variable d to the element to the head of the page - getting elements of d by their tag name that is 'head' *//*
d.getElementsByTagName('head')[0].appendChild(css);
/*This fills the array imgs with the images - taking the style slideshow and the ebement by theit tag name. The loop for modifies the img(nn) each loop adding 1 to the variable i - the image is displayed with no opacity - the maximum value fixed for the number i is 99 (99 images)*//*
imgs = d.getElementById('slideshow').getElementsByTagName('img');
for(i=1;i<imgs.length;i++) imgs[i].xOpacity = 0;
imgs[0].style.display = 'block';
imgs[0].xOpacity = .99;
setTimeout(so_xfade,4000);
}
/*the the upper bracket says that here ends the funciton SO_INIT - 4000 is the timeout of 4ª that image wait before beginning th function so_xFade */
/*The function so_xfade thatmakes the fading effect is described below here*/
function so_xfade()
{
cOpacity = imgs[current].xOpacity;
nIndex = imgs[current+1]?current+1:0;
nOpacity = imgs[nIndex].xOpacity;
cOpacity-=.05;
nOpacity+=.05;
imgs[nIndex].style.display = 'block';
imgs[current].xOpacity = cOpacity;
imgs[nIndex].xOpacity = nOpacity;
setOpacity(imgs[current]);
setOpacity(imgs[nIndex]);
if(cOpacity<=0)
{
imgs[current].style.display = 'none';
current = nIndex;
setTimeout(so_xfade,4000);
}
else
{
setTimeout(so_xfade,100);
}
function setOpacity(obj)
{
if(obj.xOpacity>.99)
{
obj.xOpacity = .99;
return;
}
obj.style.opacity = obj.xOpacity;
obj.style.MozOpacity = obj.xOpacity;
obj.style.filter = 'alpha(opacity=' + (obj.xOpacity*100) + ')';
}
} |
IMAGES SEARCH AND FITTING
Now, we have to prepare some images to put into our slideshow. Their dimensions should be 700 x 500 px. In case of different images using the appropriate image editor we can
1) change image's dimensions
- resize the original to the 700x 500px dimensions if the ratio W/H is the same
- save with name img1 or img2 or img3 .... with the appropriate number that is the order we want to show
- if the image image doesn't meet W/h add a transparent 700x500 canvas after resizing to the dimension that we want (lower than 700 x 500px. We can also crop a zone of 700xd 500px.
2) adjust colors and other parameters if you want
3) save for web your images in the appropriate folder where's easy. This can reduce the weight to a reasonable KB number to shorten the image weight. The appropriate image editor will help you to minimize trhis factor. To reduce the weight you can save to compressed jpg files. When you want canvas transparency or image transparencies, you can use png or gif files. Before saving try the different possibilities to catch tne more convenient for you.
WARNING: do not change or resize the originals. When youre saving a resized image save it with a different name. close the original and discard the candes. If you've a better method try it..
Example with 5 images downloaded and adapted
|
Images data |
Thumb height 100px |
Original |
Tower bridge 960 x 720 resolution 300 |
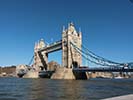 |
Thumb |
jpg 130 x 100 weight = 15,7 KB |
img1 |
jpg 700 x 500 compressed = 60 KB |
|
Original |
One thousand italian lire (today 50 cents) |
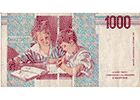 |
Thumb |
jpg 140 x 100 weight = 8KB |
img2 |
jpg 700 x 500 compressed = 68 KB |
|
Original |
Merry Christmas at home 700 x 500 288 KB |
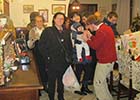 |
Thumb |
ipg 140 x 100 weight = 27 KB |
img3 |
jpg 700 x 500 compressed = 53 KB |
|
Original |
Restaurant at Albano's lake 700 x 500 300 KB |
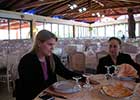 |
Thumb |
ipg 140 x 100 weight = 8 KB |
img4 |
jpg 700 x 500 compressed = 55 KB |
|
Original |
Enea and sister Stella 700 x 500 320 KB |
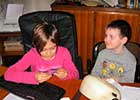 |
Thumb |
ipg 140 x 100 weight = 8 KB |
img6 |
jpg 700 x 500 compressed = 64 KB |
Original |
Carlota,Constanza,Federico,Alberto700x500-400KB |
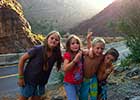 |
Thumb |
ipg 140 x 100 weight = 8 KB |
img5 |
jpg 700 x 500 compressed = 53 KB |
|
Proceedings in logical order::
- GET the images you want and oper in your image editor
- SAVE the originals into a dedicated directory (before you could also reduce the weight - if too much - by saving a compressed jpg as original)
- RESIZE OR ADAPT the image to 700x500px. Or you can also put a canvas 700x500 if the image is smaller and SAVE WITH NAME preferably in a subdir of the previous one.
- OPEN THE PREVIOUS IMAGE and compress as possible to a good image.
- SAVE WITH NAME YOUR COMPRESSED IMAGES img1 , img2, img2,......., imgn in the order tha you want to show
HTML CODE and test
Now we have to set the compressed images into the html code, remembering that their size is fixed to 700 x 500px to fit the iframe dimensions. Here is the text of html code
/*This add a so called 'event listener' that run the event when the page is loaded. starting the function so_init */
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<script type="text/javascript" src="xfade2.js"></script>
<title>My photos just for a sample of fading sequence</title>
<meta name="description" content=" Random taken photos just for a sample of fading sequence" />
<meta name="robots" content="nofollow" />
<link href="slideshow.css" rel="stylesheet" type="text/css" />
<style type="text/css">
body {
background-image: url(../../../images/roma-di-notte.jpg);
margin: 0px;
}
</style>
</head>
<body >
<div id="slideshow">
<p align="center"> <a href="../imgs/originali/tower-bridge-1426728_700_525.jpg" target="_blank"><img src="../imgs/img1.jpg" alt="Tower bridge" title="London - tower bridge 2 " width="700" height="500"/></a>
<a href="../imgs/originali/millelire.jpg" target="_blank"><img src="../imgs/img2.jpg" alt="One thousand lire" title="Italy - our beloved 1000 lire" width="700" height="495"/></a>
<a href="../imgs/originali/merrychristmasr.jpg"><img src="../imgs/img3.jpg" alt="Merry Christmas" title="Rome - merry Christmas in family" width="700" height="495"/></a>
<a href="../imgs/originali/alriistoranter.jpg" target="_blank"><img src="../imgs/img4.jpg" alt="At Albano'lake" title="Restaurant with wife and Maita - 2015 " width="700" height="495"/></a>
<a href="../imgs/originali/nipoticile.jpg" target="_blank"><img src="../imgs/img5.jpg" alt="Beautiful children" title="My chilean nephews - 2015 " width="700" height="495"/></a>
<a href="../imgs/originali/nipotitia.jpg" target="_blank"><img src="../imgs/img6.jpg" alt="Stella and Enea" title="My italian nephews - 2015 " width="700" height="495"/></a>
</div>
<script type="text/javascript">
<!--
var message="freeware2016 by Lino's page";
///////////////////////////////////
function clickIE4(){
if (event.button==2){
alert(message);
return false;
}
}
function clickNS4(e){
if (document.layers||document.getElementById&&!document.all){
if (e.which==2||e.which==3){
alert(message);
return false;
}
}
}
if (document.layers){
document.captureEvents(Event.MOUSEDOWN);
document.onmousedown=clickNS4;
}
else if (document.all&&!document.getElementById){
document.onmousedown=clickIE4;
}
document.oncontextmenu=new Function("alert(message);return false")
// --></script>
</body>
</html>
|
TIP AND TRICK (EXERCISE)
i SUGGEST A TRICK THAT PERHAPS TOU KNOW, AND MAYBE SHOULD BE VERY USEFUL TO COPY HTML PAGES THAT YOU OPEN IN YOUR BROWSER.
IF YOU WANT COPY AND ARRANGE OUR PAGE WITH FADING IMAGES, DO AS FOLLOWS.
- MAKE A DIR WHEREVER YOU WANT
- OPEN THE PAGE IN THE BROWSER CLICKING THE BUTTON AT THE END OF THE PREVIOUS PARAGRAPH (show the image)
- SAVE THE HTML PAGE INTO THE DIR YOU'VE JUST CREATED AND CLOSE THE BROWSER
- OPEN THE JUST CREATED DIR WITH YOUR WINDOW EXPLORER
|
your DIR |
|
|
__________________|__________________
|
| |
|
| |
DIR with all the files
connected to your
new html file
|
|
Your new
'saved as' html file |
|
If you before put this new named dir as a subdirectory into the root directory of your site, using the appropriate editor , you could shift each file to a more appropriate place in your site's folders structurre getting links of each file self-changed by the editor itself.
TRY SAVING THIS SAMPLE PAGE INTO A KNOWN DIR AND SEE THE SAVED FILES
|